Mastering WP_Query in WordPress is essential for developers aiming to have full control over content display. This powerful class enables the creation of customized queries, allowing for efficient and effective content delivery. Since WordPress powers approximately 43.7% of all websites, understanding and utilizing WP_Query is crucial, as it serves as the platform’s industry standard for content retrieval. This guide will explore using WP_Query for custom queries, optimizing performance, and implementing best practices to elevate your WordPress development skills.
Unlocking the Potential of Custom WordPress Queries
Creating custom queries with WP_Query allows you to dictate exactly what content to show and how to display it. This section will guide you through the essentials of crafting and leveraging custom queries to optimize your site’s content.
How to Create Your First Custom Query
Before diving in, it’s essential to understand the anatomy of a WP_Query. Here’s a simple example:
$args = array(
'post_type' => 'post',
'posts_per_page' => 5,
);
$query = new WP_Query($args);
if ($query->have_posts()){
while ($query->have_posts()) { $query->the_post(); // Output your post content here
}
wp_reset_postdata();
}
In this snippet:
post_type
: Specifies the type of content you want to retrieve, such as posts, pages, or custom post types.posts_per_page
: Limits the number of posts retrieved (5 in this example), which is critical for performance.
This example fetches five posts and loops through them using The Loop, a key part of WordPress development that you’ll use frequently.
Why Custom Queries Matter for Your WordPress Site
Custom queries aren’t just about pulling content; they’re about enhancing the user experience. By strategically organizing your content, you can:
- Improve navigation: Visitors can find relevant content faster.
- Optimize loading times: Only load what you need, when you need it.
- Create tailored experiences: Show different content to different audiences.
Understanding the mechanics of custom queries will help you deliver a more personalized, efficient site.
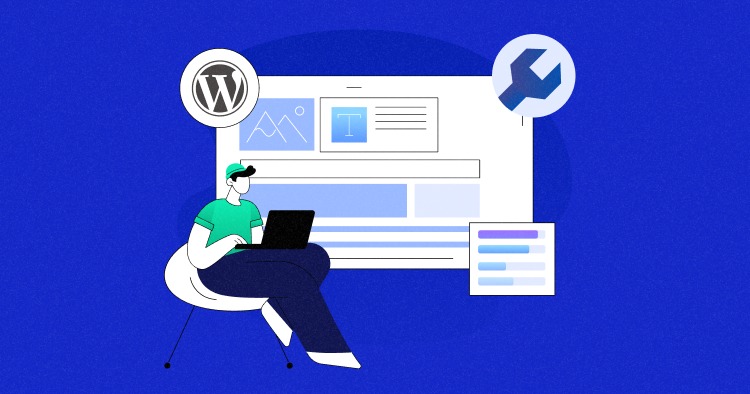
Decoding WP_Query Parameters: A Developer’s Guide
With WP_Query, you have access to a vast array of parameters that let you filter and customize the data retrieved. Let’s break down the most crucial ones and how to use them.
Must-Know WP_Query Parameters for Beginners
Every WP_Query starts with a set of parameters, which act as the building blocks of your custom query:
- post_type: Defines the type of content you’re querying, such as
post
,page
, orcustom post type
. - posts_per_page: Specifies how many posts to show.
- order and orderby: Control the order of your posts.
- category_name: Filters posts by category name.
- tag: Retrieves posts with a specific tag.
- meta_query: Allows for complex filtering using custom fields.
These parameters give you granular control over your content, making it possible to tailor your WordPress site to your exact needs.
Mixing and Matching Parameters for Ultimate Flexibility
Combining multiple parameters enables you to fine-tune your queries. Here’s a more advanced example:
$args = array(
'post_type' => 'product',
'posts_per_page' => 10,
'order' => 'ASC',
'category_name' => 'featured',
);
$query = new WP_Query($args);
In this scenario:
post_type
: Fetches content from a custom post type called “product.”posts_per_page
: Limits the result to 10 items.order
: Specifies ascending order, ideal for chronological lists.category_name
: Ensures only “featured” products are displayed.
Mastering these combinations allows you to create more refined and efficient queries.
Using WP_Query with Custom Post Types: What You Need to Know
Custom post types expand WordPress’s functionality, and WP_Query is indispensable for managing and displaying these types of content.
How to Display Custom Post Types Like a Pro
If your site relies on custom post types, you’ll need to use WP_Query to fetch and display them properly:
php
Copy code
$args = array(
'post_type' => 'portfolio',
'posts_per_page' => 3,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) { $query->the_post(); // Your custom content output } wp_reset_postdata();
}
In this code:
post_type
: Fetches posts from your “portfolio” custom post type.posts_per_page
: Limits the display to three items, ideal for a compact portfolio showcase.
Pro Tips for Handling Custom Post Types
- Proper Registration: Always register your custom post types using
register_post_type()
. This ensures that your content is queryable and accessible. - Taxonomy Filtering: Use taxonomies (custom or built-in) to further refine your content. Example:
$args = array( 'post_type' => 'portfolio', 'tax_query' => array( array( 'taxonomy' => 'project_type', 'field' => 'slug', 'terms' => 'web-design', ), ), ); $query = new WP_Query($args);
This query fetches portfolio items tagged with the “web-design” taxonomy, making it easier to organize and display your content.
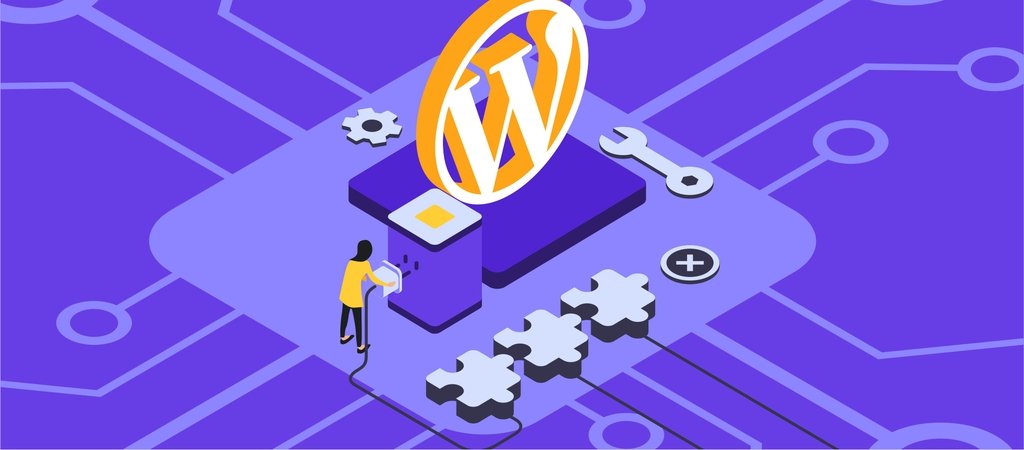
5 WP_Query Examples You Can Use Right Now
Sometimes, seeing real-world examples helps solidify your understanding. Here are two essential scenarios where WP_Query shines.
Your Go-To Example for Simple Post Lists
Fetching the latest three posts is a common requirement:
$args = array(
'posts_per_page' => 3,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post(); echo '<h2>' . get_the_title() . '</h2>'; echo '<p>' . get_the_excerpt() . '</p>';
}
wp_reset_postdata();
}
This code outputs the titles and excerpts of the latest three posts, making it easy to set up a “Latest News” or “Recent Articles” section.
Advanced Filtering: Making Complex Queries Simple
To create more sophisticated queries, combine different filters:
$args = array(
'post_type' => 'event',
'meta_query' => array(
array(
'key' => 'event_date',
'value' => date('Y-m-d'),
'compare' => '>=',
'type' => 'DATE',
),
),
'orderby' => 'meta_value',
'order' => 'ASC', );
$query = new WP_Query($args);
This query pulls upcoming events, ensuring only future dates are shown and orders them chronologically. Perfect for an event calendar!
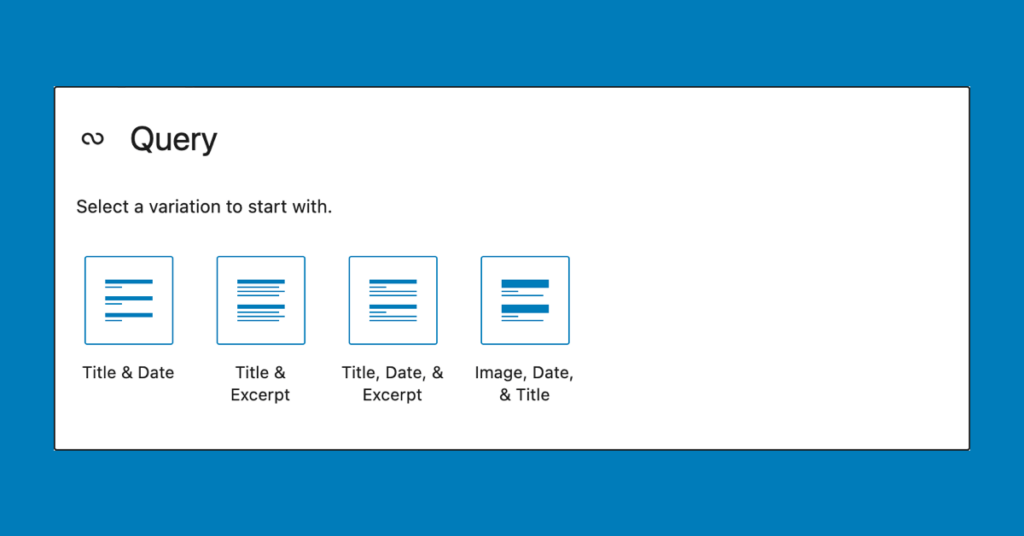
Filtering Posts with WP_Query: From Basics to Advanced
Filtering posts is a powerful way to display exactly what you need. Whether you’re sorting by category, tag, or custom field, WP_Query has you covered.
Filtering Posts by Category or Tag
Categories and tags are standard in WordPress. Here’s how to use them:
$args = array(
'category_name' => 'news',
'posts_per_page' => 5,
);
$query = new WP_Query($args);
This example fetches five posts from the “news” category, making it ideal for category-specific sections of your site.
Going Deep with Custom Field Filtering
Custom fields let you take filtering to another level:
$args = array(
'meta_query' => array(
array(
'key' => 'featured',
'value' => 'yes',
),
),
);
$query = new WP_Query($args);
This query pulls posts marked as “featured” using a custom field. It’s perfect for highlighting special content.
Making Pagination Work with WP_Query
If your site displays a lot of content, pagination ensures it remains performant and user-friendly.
Simple Steps to Add Pagination
Add the paged
parameter to your query to paginate your content:
$paged = (get_query_var('paged')) ? get_query_var('paged') : 1; $args = array( 'posts_per_page' => 10, 'paged' => $paged, ); $query = new WP_Query($args);
Using get_query_var('paged')
ensures the correct page number is retrieved from the URL.
Enhancing Navigation with Pagination Links
For complete pagination, add navigation links:
echo paginate_links(array( 'total' => $query->max_num_pages, ));
These links allow users to navigate easily between pages of content, enhancing the user experience.
Optimizing WP_Query: Tips to Keep Your Site Fast
Efficiency is crucial, especially for high-traffic websites. Let’s explore techniques to make your queries performant.
Reducing Load with Efficient Queries
Fetching too much data slows your site. Always limit the number of posts:
$args = array('posts_per_page' => 5); $query = new WP_Query($args);
Use specific filters like post_type
and meta_query
to reduce the burden on your database.
Leveraging Caching to Boost Speed
Caching saves your database from executing repeated queries. Here’s how to use transients:
$posts = get_transient('custom_query_posts');
if (false === $posts) {
$args = array('posts_per_page' => 5);
$query = new WP_Query($args);
set_transient('custom_query_posts', $query->posts, 12 * HOUR_IN_SECONDS); }
By caching query results for 12 hours, you significantly reduce server load.
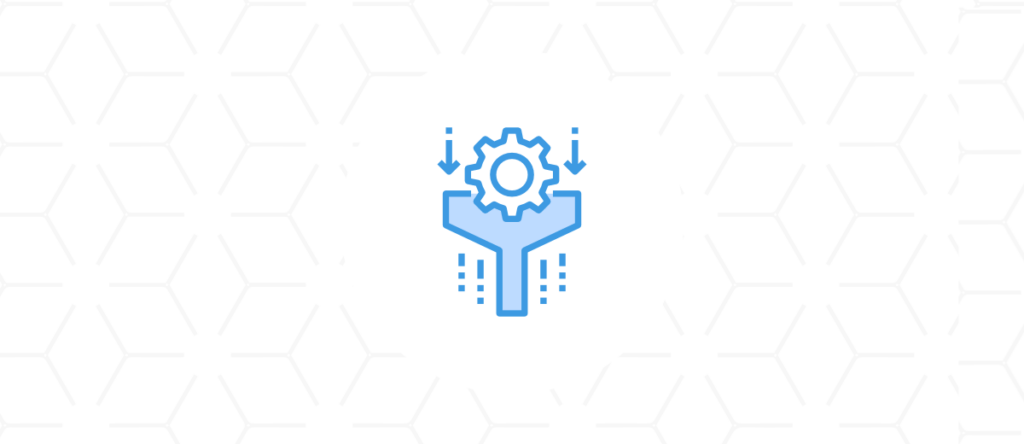
Mastering the pre_get_posts Hook for Ultimate Control
The pre_get_posts
hook allows you to modify the main query before it runs, providing unparalleled control over content retrieval.
How to Customize Queries with pre_get_posts
Use this hook to change what content is displayed on specific pages:
function modify_main_query($query) {
if (is_home() && $query->is_main_query()) {
$query->set('posts_per_page', 5);
}
}
add_action('pre_get_posts', 'modify_main_query');
This function modifies the main query on the homepage to show only five posts.
Creative Use Cases for pre_get_posts
The pre_get_posts
hook is highly versatile. You can use it to:
- Filter search results to include only posts, not pages.
- Modify custom post type archives to display in a specific order.
- Limit posts on category archives for better performance.
WP_Query vs. get_posts vs. query_posts: What’s the Difference?
Choosing the right function to query posts in WordPress can significantly impact your site’s performance and functionality. Understanding the differences between WP_Query, get_posts, and query_posts will help you make informed decisions when working with custom content retrieval.
Why WP_Query Is the Preferred Choice
WP_Query is the most powerful and flexible method for querying posts in WordPress. It allows you to create custom queries tailored to your exact needs without interfering with the main global query. This makes it ideal for scenarios where you need to control the content output precisely.
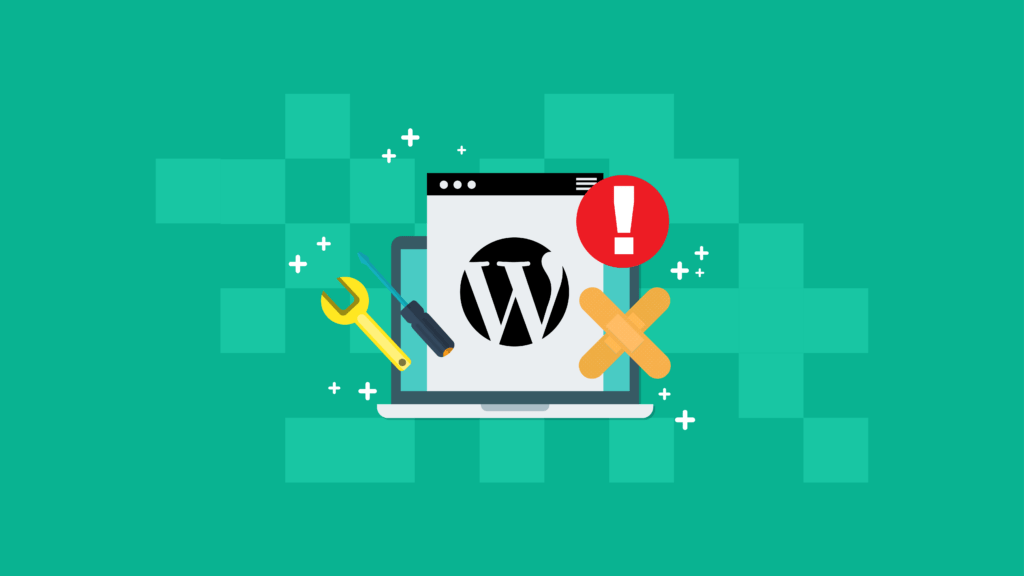
Potential Pitfalls and Solutions of WP_Query
Even though WP_Query is robust, developers often encounter pitfalls that can lead to significant site issues. Fortunately, there are strategies to resolve these problems effectively.
1. Resetting the Post Data
One of the most common mistakes is forgetting to reset the post data after running a custom WP_Query loop. If you don’t reset the global $post
object, it can cause unexpected behavior in other loops or parts of your site that rely on the default query.
- Problem: When you use
the_post()
inside a custom loop, the global$post
object is modified. If you don’t reset this data, other queries, such as the main blog feed or widgets, might display incorrect posts. - Solution: Always use
wp_reset_postdata()
at the end of your custom loop. This function restores the global$post
object to its original state, ensuring that subsequent queries behave as expected.
Example:
Mind Your Business Newsletter
Business news shouldn’t put you to sleep. Each week, we deliver the stories you actually need to know—served with a fresh, lively twist that keeps you on your toes. Stay informed, stay relevant, and see how industry insights can propel your bottom line.
Subscribe to the newsletter
$args = array(
'posts_per_page' => 5,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) { $query->the_post(); // Custom loop content
}
wp_reset_postdata(); // Reset post data
}
By resetting the post data, you ensure that your main query or other custom queries are not affected.
2. Storing the Global Post Object
Another workaround to prevent issues with the global $post
object is to store it in a temporary variable before running your custom loop. This approach allows you to restore the $post
object manually, providing more control over the data flow.
- Problem: In some cases, even when using
wp_reset_postdata()
, you may want additional control over the global post object to ensure it retains its original state. - Solution: Store the global
$post
object in a temporary variable before modifying it and restore it afterward.
Example:
global $post;
$original_post = $post; // Store the global post object
$args = array(
'posts_per_page' => 5,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) { $query->the_post(); // Custom loop content
}
$post = $original_post; // Restore the global post object setup_postdata($post); }
This approach is especially useful when dealing with complex templates where multiple loops are used, and data consistency is critical.
Avoiding Pitfalls with query_posts
While query_posts
is often used for modifying the main query, it comes with significant drawbacks that can impact site performance and functionality.
- Problem:
query_posts
overrides the main query and re-runs the database query, which can lead to performance degradation, especially on pages with multiple queries. It can also interfere with pagination and other default behaviors. - Solution: Avoid using
query_posts
. Instead, modify the main query safely using thepre_get_posts
hook or use WP_Query for custom queries.
Example of the pre_get_posts Hook:
function modify_main_query($query) {
if (!is_admin() && $query->is_main_query() && is_home()) {
$query->set('posts_per_page', 10);
}
}
add_action('pre_get_posts', 'modify_main_query');
Using pre_get_posts
is a safer and more efficient way to alter the main query without the performance issues associated with query_posts
.
Understanding get_posts
get_posts is a simplified version of WP_Query that fetches an array of post objects. It’s useful for situations where you need a quick, read-only query without the full capabilities of WP_Query.
- Use Case: Use
get_posts
for simple queries where performance is crucial, such as fetching a list of posts for a sidebar or a footer widget. - Limitation:
get_posts
lacks the full flexibility of WP_Query and does not use The Loop, making it less suitable for scenarios that require advanced querying.
Example:
$args = array(
'numberposts' => 5,
'category' => 3,
);
$recent_posts = get_posts($args);
foreach ($recent_posts as $post) {
setup_postdata($post); echo '<h2>' . get_the_title() . '</h2>';
}
wp_reset_postdata();
Summary: Use WP_Query for full control and flexibility, get_posts for lightweight queries, and avoid query_posts
whenever possible. Understanding these differences will help you optimize your site’s performance and functionality.
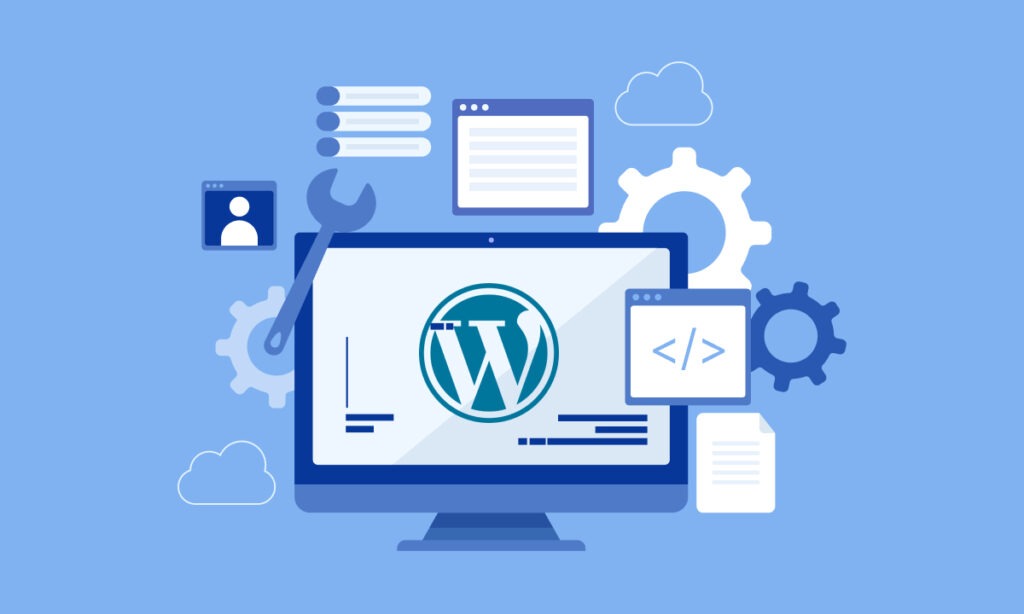
Resetting WP_Query: A Crucial Step You Can’t Skip
Failing to reset WP_Query can lead to conflicts, especially when working with global variables.
wp_reset_postdata vs. wp_reset_query Explained
When using custom queries, always reset post data to maintain site integrity:
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post(); // Output your content
}
wp_reset_postdata();
}
- wp_reset_postdata(): Resets global post data to ensure subsequent queries work as expected.
- wp_reset_query(): Resets the main query, but it should only be used in specific situations.
WP_Query Examples: Ready-to-Use Code Snippets
Understanding WP_Query becomes even more intuitive when you see it in action. Below are several pre-made examples that incorporate essential parameters such as loops, author information, categories, date queries, and more. Each example is followed by an explanation to help you implement these queries effectively.
Example 1: Display Posts by a Specific Author in Descending Order
This example showcases how to use the WP_Query class to fetch posts written by a specific author, ordered by date in descending order.
$args = array( 'author' => 2, // Replace 2 with the author's ID 'posts_per_page' => 5, 'orderby' => 'date', 'order' => 'DESC', ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); echo '<h2>' . get_the_title() . '</h2>'; echo '<p>Written by: ' . get_the_author() . '</p>'; } wp_reset_postdata(); }
Explanation:
- author: Filters posts by the author’s ID. Replace
2
with the desired author ID from your WordPress database. - orderby: Orders the posts by the publication date.
- order: Specifies descending order (
DESC
), showing the latest posts first. - wp_reset_postdata(): Ensures that global post data is reset after the custom query loop.
Example 2: Query Posts from a Specific Category with Author Information
Here’s how to display posts from a specific category, along with the author’s name and a custom excerpt.
$args = array(
'category_name' => 'technology', // Replace with your category slug
'posts_per_page' => 3,
'orderby' => 'title',
'order' => 'ASC',
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post(); echo '<h2>' . get_the_title() . '</h2>'; echo '<p>By: ' . get_the_author() . '</p>'; echo '<p>' . get_the_excerpt() . '</p>';
}
wp_reset_postdata();
}
Explanation:
- category_name: Filters posts by the category slug, such as “technology.”
- orderby: Sorts posts alphabetically by title (
title
). - order: Specifies ascending order (
ASC
), useful for a directory-style listing. - The loop outputs the post title, author name, and an excerpt.
Example 3: Fetch Posts Using a Date Query in Descending Order
Use this example to pull posts published after a certain date, ordered by most recent first.
$args = array(
'date_query' => array(
array(
'after' => 'January 1, 2023',
'inclusive' => true,
),
),
'posts_per_page' => 5,
'orderby' => 'date',
'order' => 'DESC',
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) { $query->the_post(); echo '<h2>' . get_the_title() . '</h2>'; echo '<p>Published on: ' . get_the_date() . '</p>'; } wp_reset_postdata();
}
Explanation:
- date_query: Filters posts published after a specified date (
after
parameter). Theinclusive
flag includes posts published on the exact date. - orderby: Uses the date to order posts.
- order: Descending order (
DESC
) ensures that newer posts appear first. - This query is ideal for displaying recent content since a specific date.
Example 4: Retrieve Posts in a Custom Order with Multiple Parameters
Combine multiple parameters to create a highly customized query.
$args = array(
'post_type' => 'post',
'posts_per_page' => 10,
'orderby' => array(
'author' => 'ASC',
'date' => 'DESC',
),
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) { $query->the_post(); echo '<h2>' . get_the_title() . '</h2>'; echo '<p>Author: ' . get_the_author() . ' | Published on: ' . get_the_date() . '</p>';
}
wp_reset_postdata(); }
Explanation:
- orderby: An array used to order posts first by the author in ascending order (
ASC
), then by date in descending order (DESC
). - posts_per_page: Limits the number of posts to 10, optimizing the query for performance.
- This query is excellent for content that requires both author and date ordering.
Example 5: Query Posts with a Category and Date Constraint
This final example demonstrates a query that filters posts by category and only shows posts published before a specific date.
$args = array(
'category_name' => 'news',
'date_query' => array(
array(
'before' => 'December 31, 2023',
'inclusive' => true,
),
),
'posts_per_page' => 7,
'order' => 'DESC',
);
$query = new WP_Query($args);
if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); echo '<h2>' . get_the_title() . '</h2>'; echo '<p>Published: ' . get_the_date() . '</p>';
}
wp_reset_postdata();
}
Explanation:
- category_name: Restricts posts to the “news” category.
- date_query: Only includes posts published before December 31, 2023.
- order: The descending order (
DESC
) ensures the most recent posts within the date range are displayed. - Useful for archiving past content or creating a historical news section.
These examples should give you a solid foundation for using WP_Query to customize content retrieval from the WordPress database. By understanding and experimenting with these parameters, you can create highly efficient and tailored queries for any WordPress site.
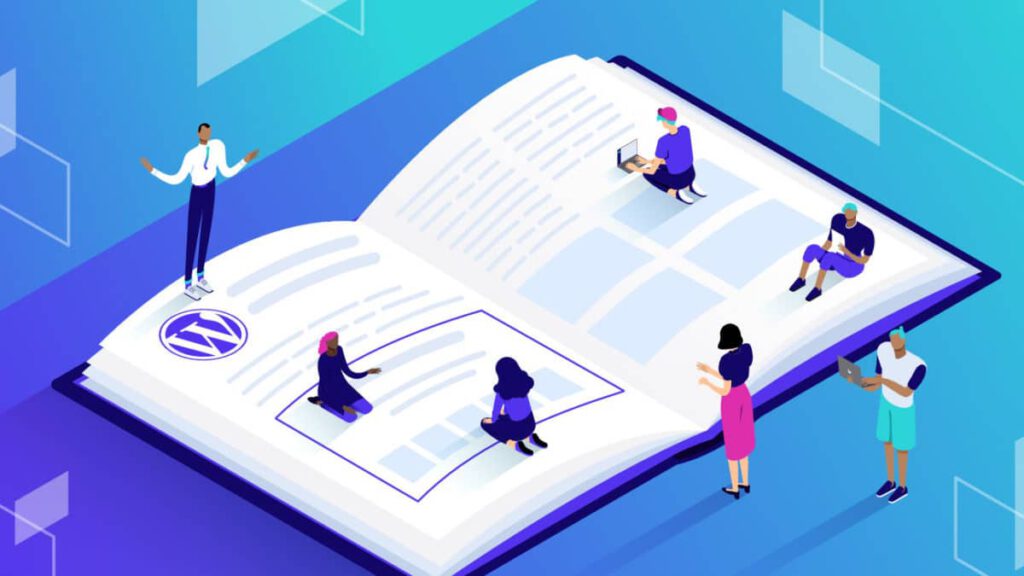
Wrapping Up How to Use WP_Query in WordPress
Understanding how to effectively use WP_Query in WordPress can transform your site’s functionality and user experience. From creating custom queries to optimizing performance, mastering these techniques is essential for any WordPress developer. Remember, the key to success lies in choosing the right query method and avoiding common pitfalls to ensure your site runs smoothly.
If you’re ready to take your WordPress customization to the next level or need professional help implementing these techniques, visit our WordPress Customization Page and discover how we can transform your website into a high-performing, user-friendly platform.